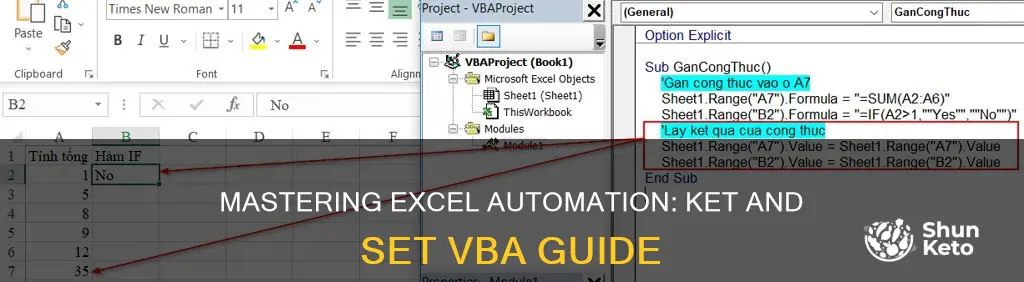
Visual Basic for Applications (VBA) is a programming language developed by Microsoft to automate repetitive tasks, enhance functionality, and create custom solutions within Microsoft Office applications like Excel, Word, and Access. VBA allows users to write scripts or small programs to perform tasks such as data manipulation, complex calculations, and generating reports, making work more efficient and customized to specific needs.
VBA Class Modules are a type of VBA code that allows users to create their own objects and build applications like they are using building blocks. The code for each object is self-contained and independent of any other code in the application, making it easier to test and update code without causing problems in other parts of the application.
To set a cell value in VBA, you can use the Range or Cells function. The Range function helps access cells in a worksheet, while the Cells function is similar but can only set the value of a single cell at a time. By using these functions, you can set the value of a single cell or multiple cells simultaneously.
Additionally, VBA has properties such as Get, Set, and Let that allow you to interact with cell values. The Get property is used to retrieve the value of a cell, the Set property is used to assign a value to a cell, and the Let property is used to modify an existing value in a cell.
Overall, VBA and its Class Modules provide users with powerful tools to automate tasks, improve efficiency, and customize their Excel experience.
Characteristics | Values |
---|---|
Purpose | Automate repetitive tasks, enhance functionality, and create custom solutions within Microsoft Office applications like Excel, Word, and Access |
Language | Visual Basic for Applications (VBA) |
Use | Write scripts or small programs to perform tasks such as data manipulation, complex calculations, and generating reports |
Cell Value Reference | Range Object ("A1".Value) or Cells Object (2,1).Value |
Cell Value Types | Numerical or Text |
Multiple Cell Values | Reference a range of cells and change all values at once |
Variable Cell Value | Dim strText as String strText = "String of Text" Range("A2").Value = strText |
Active Cell Value | MsgBox ActiveCell.Value |
Assign Cell Value to Variable | Dim var as Variant var = Range("A1").Value |
What You'll Learn
Using the Range function to set a single cell value
Syntax: The basic syntax for setting the value of a single cell using the Range function is as follows:
Range("cell_address").Value = value_to_assign
- Specifying the Cell Address: In the code, you need to specify the address of the cell you want to modify. For example, to set the value of cell A1, you would use "A1" as the cell address.
- Assigning the Value: You can assign different types of values to the cell. This can be a static value, such as a number or text, or it can be a variable. Here are some examples:
Range("A1").Value = 10 ' Assigning a numeric value
Range("B2").Value = "Hello" ' Assigning a text value
Dim myValue as String
MyValue = "World"
Range("C3").Value = myValue ' Assigning a variable value
Running the Macro: Once you have defined the code to set the cell value, you can run your macro. This will execute the code and set the specified cell to the assigned value.
Remember that when using the Range function, you are directly modifying the value of the cell in the worksheet. This means that any changes made using VBA will be immediately reflected in the Excel sheet.
Keto Cooking: Calculating Your Customized Carb-Free Creations
You may want to see also
Using the Range function to set multiple cell values at once
Syntax: The basic syntax for setting values in multiple cells using the Range function is as follows:
Range("Start_Cell:End_Cell").Value = "Value_to_be_assigned"
- Specifying the Range: To specify the range of cells, use the "Start_Cell:End_Cell" format. For example, to set values in cells A2 to A11, you would use "A2:A11".
- Assigning the Value: After specifying the range, you can assign the desired value to the selected cells. For instance, if you want to set the text "Arushi" in cells A2 to A11, your code would look like this:
Range("A2:A11").Value = "Arushi"
Running the Macro: Once you've set the range and assigned the value, simply run your macro. In this example, the text "Arushi" will appear in cells A2 to A11.
By using the Range function, you can efficiently set the same value in multiple cells with just one line of code, making it a powerful tool for automating tasks in Excel.
Keto Coach Meter: Your Ultimate Guide to Using It
You may want to see also
Using the Cells function to set a single cell value
To set a single cell value in Excel VBA, you can use the Cells function. The Cells function is used to set the cell value in a worksheet and uses a matrix coordinate system to access cell elements.
Let's say you want to set the value of cell B1 to "Arushi cleared CA with Rank". Here are the steps:
- Open your VBA editor.
- Use the Cells function, specifying the row and column number of the cell you want to access. In this case, since we want to access cell B1, the matrix coordinates will be (1, 2).
- Type the following code into your VBA editor:
Vb
Cells(1, 2).Value = "Arushi cleared CA with Rank"
Run your macro.
Now, the text "Arushi cleared CA with Rank" will appear in cell B1.
You can also set the value of a cell without using the `.Value` property by entering the following code:
Vb
Cells(1, 2) = "Arushi cleared CA with Rank"
However, it is recommended to use the `.Value` property when entering a value into a cell.
Additionally, if you want to enter a number into a cell, you do not need to use quotation marks. For example, to set the value of cell A1 to the number 11, you can use the following code:
Vb
Range("A1").Value = 11
The Cells function is similar to the Range function, which can also be used to set cell values. The difference is that the Cells function can only set a single cell value at a time, while the Range function can set multiple cell values simultaneously.
Mastering Brace and Kets: A Comprehensive Guide
You may want to see also
Using the ActiveCell function to set a cell value
The ActiveCell function in VBA for Excel is used to refer to the currently selected cell in a workbook. It is a property that represents the cell that is active at the moment.
To use the ActiveCell function, follow these steps:
- Select a cell or navigate to a cell so that the green box covers it.
- Type "ActiveCell" in your VBA code.
- Type a dot (.) to get a list of properties and methods that you can use with ActiveCell.
- Select the desired property or method, such as .Value or .Address.
- Run the code to perform the desired activity on the active cell.
For example, the following code will return the value from the active cell using a message box:
Vb
MsgBox ActiveCell.Value
You can also set the value of the active cell using the ActiveCell function:
Vb
ActiveCell.Value = value_to_be_assigned
Vb
Sub vba_activecell()
ActiveCell.Value = "Arushi is practicing CA"
ActiveCell.Interior.Color = vbYellow
End Sub
Note that you can only work with the active cell when the worksheet it is on is the active sheet. To activate a specific worksheet, use the following code:
Vb
Worksheets("Sheet1").Activate
The ActiveCell function is a powerful tool in VBA for Excel that allows you to automate tasks and manipulate data in the active cell of a workbook.
Keto Salts: How Frequently Should You Consume Them?
You may want to see also
Using the Input Box to set a cell value
Understanding the InputBox Function: The InputBox function in VBA allows you to prompt the user to enter a value. This value can be text, a number, or any other type of input. The basic syntax for the InputBox function is:
InputBox("Prompt Text", "Title", "Default Value")
Here, "Prompt Text" is the message displayed in the input box, "Title" is the title of the input box, and "Default Value" is an optional value that appears in the input box initially.
Declaring a Variable: Before using the InputBox, you should declare a variable to store the user's input. Typically, you would use a Variant variable because it can hold any type of value. Here's an example:
Dim userInput As Variant
Displaying the Input Box: To show the input box to the user, you can use the following code:
UserInput = InputBox("Enter a value:", "Input Box Title", "")
This code will display an input box with the prompt "Enter a value:", a title of "Input Box Title", and no default value.
Setting the Cell Value: Once you have the user's input stored in the variable, you can set the value of a specific cell using the Range or Cells function. For example:
Range("A1").Value = userInput
Or:
Cells(1, 1).Value = userInput
This code will set the value of cell A1 to the value entered by the user in the input box.
Running the Macro: After setting the cell value, you can run your macro to see the results. The specified cell (in this case, A1) will now contain the value entered by the user in the input box.
Using the Input Box is a flexible way to get user input and set cell values in VBA. It allows users to provide different types of input, and you can customize the appearance and behavior of the input box to suit your needs.
Keto Fast: Effective Weight Loss Strategies
You may want to see also
Frequently asked questions
To set the value of a specific cell, you can use the following code:
```
Range("A1").Value = "Your Value"
```
This sets the value of cell A1 to "Your Value".
To get the value of a specific cell, you can use this code:
```
Dim cellValue As String
cellValue = Range("A1").Value
```
This stores the value of cell A1 in the variable cellValue.
You can use an If statement to change a cell value based on a condition:
```
If Range("A1").Value = "Old Value" Then
Range("A1").Value = "New Value"
```
This changes the value of cell A1 to "New Value" if it currently contains "Old Value".
You can set the value of a cell using a variable like this:
```
Dim newValue As String
newValue = "Hello, Excel!"
Range("B2").Value = newValue
```
This sets the value of cell B2 to the value stored in newValue.